Being an Android developer, you must want to know how to use Zxing that is an Android QR code scanner library. This library helps to read QR code. In this tutorial, we will learn how to use this library to read the QR Code in the Android app. So, let’s get started!
Table of Contents
What is ZXing?
ZXing refers to as a “Zebra Crossing,” which is an open-source library for barcode scanning and generation. It supports various formats, including QR codes and 1D/2D barcodes. Developed by Google, it is widely used in mobile apps for reading and generating codes using device cameras.
What is QR code?
After barcodes, QR codes have become universal in the past few years. You might have also noticed advertisements and/or certain brand products. In simple terms, QR codes are images or data matrix that are designed to be read by all machines, just like the barcodes. Both QR codes in Android and iOS represent a small string like a shortened web URL and/or a contact number.
Here’s a QR code example that contains URL of Space-O Technologies.
Unlike barcodes, the QR codes can be scanned accurately with a smartphone by giving the camera permission to scan. And it doesn’t require any specialized hardware like the barcodes. However, in some smartphones, you may need a QR code scanner for accessing QR codes.
In this tutorial, we will learn how to read QR code with Android by using the android QR scanner library and zxing. But before we get started, let’s first understand the QR code.
Understanding QR Code
QR code or Quick Response code is basically a two-dimensional barcode that decodes contents at a higher speed. The first QR code system was invented by a Japanese company called Dense-Wave in 1994.
And today, even a smartphone having a barcode reader app can scan and decode any QR code. Therefore in this Android barcode scanner library tutorial, we’re going to demonstrate the process of scanning the image of QR code Android at the click of a button using the Zxing library.
Before we start this tutorial, don’t miss checking the QR scanner app developed by our app developers.
Let’s Develop Your QR Scanner App Idea Together
Get in touch with us to build a QR scanner app that can help your business streamline operations and enhance customer experience.
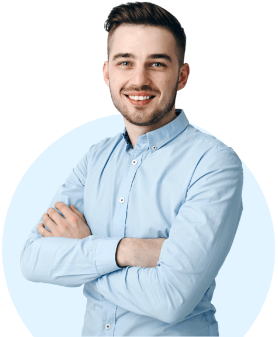
Steps to Read QR Code in Android Using Zxing Library
First of all, create a new project in Android Studio.
Then, add aar dependency with Gradle.
Add the following to your build.gradle file: repositories { jcenter() } dependencies { compile 'com.journeyapps:zxing-android-embedded:3.4.0' compile 'com.android.support:appcompat-v7:23.1.0' // Version 23+ is required } android { buildToolsVersion '23.0.2' // Older versions may give compile errors }
Next, use IntentInegrator.
At last, launch the intent with the default options:
Start Activity For Result
new IntentIntegrator(this) .initiateScan(); // `this` is the current Activity
Get and Handle Result
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { IntentResult result = IntentIntegrator.parseActivityResult(requestCode, resultCode, data); if (result != null) { if (result.getContents() == null) { // cancel } else { // Scanned successfully { { else { super.onActivityResult(requestCode, resultCode, data); } }
Adding Customization Options
Set params
IntentIntegrator integrator = new IntentIntegrator(this); integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES); integrator.setPrompt("Scan a barcode"); integrator.setCameraId(0); // Use a specific camera of the device integrator.setBeepEnabled(false); integrator.setBarcodeImageEnabled(true); integrator.initiateScan();
Changing The Orientation
Add Activity in AndroidManifest.xml
<activity android:name="com.journeyapps.barcodescanner.CaptureActivity" android:screenOrientation="portrait" tools:replace="screenOrientation" />
Set params in Intent
IntentIntegrator integrator = new IntentIntegrator(this); integrator.setOrientationLocked(false); integrator.initiateScan();
Add Following in String.xml
<string> name="scan">Scan</string> <string> name="scan">Scan</string>
Start Code Integration
MainActivity.java
public class MainActivity extends AppCompatActivity implements OnClickListener { private Button scanBtn; private TextView tvScanFormat, tvScanContent; private LinearLayout llSearch; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); scanBtn = findViewById(R.id.scan_button); tvScanFormat = findViewById(R.id.tvScanFormat); tvScanContent = findViewById(R.id.tvScanContent); llSearch = findViewById(R.id.llSearch); scanBtn.setOnClickListener(this); } public void onClick(View v) { llSearch.setVisibility(View.GONE); IntentIntegrator integrator = new IntentIntegrator(this); integrator.setPrompt("Scan a barcode or QRcode"); integrator.setOrientationLocked(false); integrator.initiateScan(); // Use this for more customization // IntentIntegrator integrator = new IntentIntegrator(this); // integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES); // integrator.setPrompt("Scan a barcode"); // integrator.setCameraId(0); // Use a specific camera of the device // integrator.setBeepEnabled(false); // integrator.setBarcodeImageEnabled(true); // integrator.initiateScan(); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { IntentResult result = IntentIntegrator.parseActivityResult(requestCode, resultCode, data); if (result != null) { if (result.getContents() == null) { llSearch.setVisibility(View.GONE); Toast.makeText(this, "Cancelled", Toast.LENGTH_LONG).show(); } else { llSearch.setVisibility(View.VISIBLE); tvScanContent.setText(result.getContents()); tvScanFormat.setText(result.getFormatName()); } } else { super.onActivityResult(requestCode, resultCode, data); } } }
Activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.qrcodescanner.MainActivity"> <Button android:id="@+id/scan_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="@string/scan" /> <LinearLayout android:id="@+id/llSearch" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/scan_button" android:layout_marginTop="20dp" android:orientation="vertical" android:visibility="gone"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:minEms="4" android:text="Format :" android:textColor="@color/colorPrimary" android:textIsSelectable="true" android:textSize="16dp" android:textStyle="bold" /> <TextView android:id="@+id/tvScanContent" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_marginLeft="10dp" android:layout_weight="1.0" android:textColor="@android:color/black" android:textIsSelectable="true" android:textSize="14sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:orientation="horizontal"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:minEms="4" android:text="Content :" android:textColor="@color/colorPrimary" android:textIsSelectable="true" android:textSize="16dp" android:textStyle="bold" /> <TextView android:id="@+id/tvScanFormat" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_marginLeft="10dp" android:layout_weight="1.0" android:textColor="@android:color/black" android:textIsSelectable="true" android:textSize="14sp" /> </LinearLayout> </LinearLayout> </RelativeLayout>
Congratulations!
If you’ve followed the exact steps, then you’ll successfully integrate a QR code scanner in an Android app. However, if you face any problem implementing it, you can contact our Android developers for help. Here, we have tried to answer a few of the most frequently asked questions by our readers.
Frequently Asked Questions
What is the ZXing barcode scanner?
ZXing is a barcode image processing library implemented in Java, with ports to other languages.
What is a barcode library?
Barcode SDK is a software library used to add barcode features to desktop, web, mobile, or embedded applications.
How do barcode scanning work in Android?
An Android phone can scan a barcode or QR code with the help of a QR code scanning app. There are several such apps available on the Google play store.
Conclusion
Even though this was just a simple QR code reader tutorial, but you can also add certain features like automatically saving URLs and/or contact numbers on your smartphone. And in case, if you have any queries about how to scan a QR code on android or you need a technical expert like an Android developer to help you build an Android application, contact us to hire Android developer.
Grab a free copy of the QR Code Android example from Github.