Table of Contents
What is Notification Manager in Android?
Notification Manager in Android is a system service responsible for handling and displaying app notifications. It manages the notification lifecycle, ensuring a consistent and efficient user experience across different devices and Android versions.
What Generally Goes in an Android App Notification?
The most simple notifications must contain the following.
An Icon: Almost every Android phone user is busy and is always on the go. In fact, there’s no guarantee that the users will even have time to read your notification’s text.
Therefore, users must be able to recognize your app’s notifications at a glance, from the icon alone.
For this reason, you may choose your android app’s icon for your notifications, but occasionally, you can also use a different image. For example, if you’re developing an instant messaging app, you can use the sender’s profile pic instead of your app’s icon.
Create images that you want to use and add them to your project’s drawable folder.
Title Text: You can either set your notification’s title by referencing a string resource, or by adding the text to your notification directly.
Detail Text: This is the most important part of notification in Android app, therefore this text part must include everything a user needs to understand what exactly the user is being notified. However, since most mobile users are in a rush, so keep this detailed text short and snappy.
Like everything in the mobile platform, notification in Android has also evolved over time. There are lots of another notification setting you can use, although some of them are quite difficult to integrate if you’re a beginner, therefore you may want to consult with an Android application development company to add them in your Android app correctly.
Now, since you know basics about Android app notification, it’s time to learn how to add one!
Creating Your Android Notification Tutorial
Add dependency in the build.gradle file
implementation 'com.android.support:appcompat-v7:28.0.0' implementation 'com.android.support.constraint:constraint-layout:1.1.3'
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:text="Notify" android:textAllCaps="false" android:layout_width="200dp" android:layout_height="wrap_content" android:background="@drawable/shape_button" android:id="@+id/btnNotify" android:textColor="@android:color/white" android:textSize="18dp" app:layout_constraintTop_toTopOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintBottom_toBottomOf="parent"/> </android.support.constraint.ConstraintLayout>
activity_notifymessage.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.spaceo.notificationalertdemo.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent"/> </android.support.constraint.ConstraintLayout>
MainActivity
package com.spaceo.notificationalertdemo import android.app.Notification import android.app.NotificationChannel import android.app.NotificationManager import android.app.PendingIntent import android.content.Context import android.content.Intent import android.os.Build import android.os.Bundle import android.support.v4.app.NotificationCompat import android.support.v7.app.AppCompatActivity import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { private val NOTIFY_ME_ID = 1337 private val NOTIFICATION_CHANNEL_ID = "My Channel" override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // After Click On Notification Jump To NotifyMessage val notificationIntent = Intent(this, NotifyMessage::class.java) // PendingIntent Create val pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent, 0) // Notification Builder Create val builder = NotificationCompat.Builder(this@MainActivity, NOTIFICATION_CHANNEL_ID) // Custom Notification Create builder.setSmallIcon(R.drawable.notification) .setContentTitle(resources.getString(R.string.tvTitleofnotification)) .setContentText(resources.getString(R.string.tvBodyofNotification)) .setContentIntent(pendingIntent) builder.notification.flags = builder.notification.flags or Notification.FLAG_AUTO_CANCEL builder.setAutoCancel(true) // NotificationManager Create val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager // If API level 26 or higher than create channel for Notification if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { // channel Create val notificationChannel = NotificationChannel( NOTIFICATION_CHANNEL_ID, resources.getString(R.string.app_name), NotificationManager.IMPORTANCE_DEFAULT ) notificationManager.createNotificationChannel(notificationChannel) } // Button Click After Generate Notification btnNotify.setOnClickListener { notificationManager.notify(R.drawable.notification, builder.build()) } } }
Want to Develop an Android Application?
Looking to develop an Android app? Get in touch with our experienced Android app developers for a free consultation.
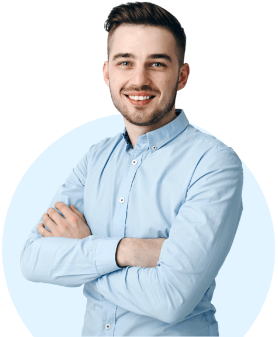
NotifyMessage
package com.spaceo.notificationalertdemo import android.os.Bundle import android.support.v7.app.AppCompatActivity class NotifyMessage : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_notifymessage) } }
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.spaceo.notificationalertdemo"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".NotifyMessage"></activity> </application> </manifest>
And Done! Here is the Output:
Now of course, in this Android app tutorial, we’ve only covered the basics of creating and employing notification in Android app. But, depending on your app and functionalities of your app, you can go much deeper while making sure to be thoughtful when using this powerful tool.
Grab a free copy of Android Notification Example from Github.