When developing an iOS app, every programmer aims for a neat and clean code structure that is easy to read, understand, and modify later. However, with thousands of lines of code, maintaining optimal readability and understandability often comes rarely.
To tackle this problem, iOS developers are now embracing clean architecture principles. By adopting clean architecture in iOS development, programmers bifurcate the entire code into distinct layers. Each of these layers separates the codebase by concerns or use.
Being an experienced iOS app development company, we have valuable experience in implementing a clean architecture for iOS projects. In this blog, we have comprehended all this information to guide you through the essentials of clean architecture in iOS app development.
Contents
5 Reasons to Use Clean Architecture in iOS App Development
Why do developers need to care about software architectures? Here are a few reasons:
- Without a systematic architecture, a program would be a disoriented set of codes and commands that are hard to work through.
- By implementing clean architecture philosophies to your iOS development project, you can streamline your code repository with managed dependencies.
- With almost 2 million apps on the App Store (as per Apple’s report), a clean architecture is what will differentiate your app from the competitors.
- Your program will stand out in terms of manageability and upgradability.
- Adding new features becomes a lot easier when the source code is easy to understand.
“I’ve used Clean Architecture in a couple of apps and it’s proven to be a valuable pattern for developing scalable, consistent, and composable architectures. I’ve also built many apps that didn’t need the complexity of Clean Architecture and I don’t feel it was wrong to not use it there.”
– Sean G. WrightLead Product Evangelist at Kentico
However, there’s more to clean architecture than easy-to-read codes. Let’s take a look at some noteworthy advantages of clean iOS architectures.
5 Key Features of a Clean iOS App Architecture
While there are numerous software architecture principles, Robert C. Martin’s clean architecture philosophy stands out for 5 main reasons. Codes written in clean architecture are modular, reusable, scalable, readable, and collaborative. Let’s understand each feature in detail.
Modular
iOS apps made using clean architecture principles have modular components. The entire app is divided into four key layers- the domain layer, the application layer, the infrastructure layer, and the presentation layer. This modular separation of layers enables iOS developers to make changes in one layer with minimal impact on the other.
Reusable
Implementing clean architecture principles allows for code reuse across different parts of the iOS application. Since the codebase is separated by concerns, it is easy to create independent components of code that can be used again and again.
Scalable
A clean iOS architecture breaks the app into different layers, hence promoting clean coding. This way, the developers can add new features and functionalities to the code easily, and that too without disrupting other layers of the program.
Readable
One of the most noteworthy characteristics of a clean architecture in iOS is easy code readability. This architectural pattern has a comprehensive view. Different components of the code are stored in predefined folders, eliminating code clutter and promoting ease of understanding.
Collaborative
Clean architecture offers iOS developers a collaborative environment to work with. With a clear separation of concerns, the front-end and back-end teams can work parallelly. Moreover, multiple developers can work on the same codebase easily due to a concise and intuitive code pattern.
Implementing clean architecture principles in iOS app development has these benefits. Now, let’s examine the layers of clean architectural patterns.
Are You Looking for a Skilled Software Architect?
Space-O Technologies brings you the finest software architecture managers with proven experience in implementing clean architecture in iOS.
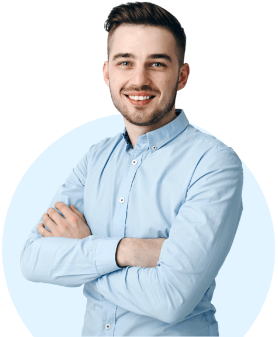
Layers of a Clean iOS Architecture
An iOS program made with the clean architecture philosophy is divided into 4 layers- domain, application, infrastructure, and presentation.
Domain Layer
- Also known as the entities layer, the domain layer is the centre-most layer of the iOS program.
- The domain layer stores all the core business logic, entities, and business rules of the application.
- This layer acts as the foundation of the application and governs the core concepts and behavior of the domain.
- The domain layer is independent and isolated and is not bound to any technology or framework.
Application Layer
- The application layer is also known as the use cases layer or interactors layer and includes business rules and use cases specific to the application.
- The application layer handles high-level business logic and executes use cases.
- Each use case is a functionality that your iOS app can perform to achieve the desired goals.
- Executing a use case will coordinate the flow of data between entities to achieve an objective.
- It is the application layer that separates the concerns of the domain layer and the interface layer.
Infrastructure Layer
- The infrastructure layer contains all the external dependencies and services that are required to run the iOS application.
- The infrastructure layer provides a solid mechanism to implement the user interfaces defined in the interface layer.
- The components of the infrastructure layer handle tasks like logging, data storage, caching, network communication, and more.
- Clean architecture in iOS enables the infrastructure layer to remain easily upgradable and replaceable.
Presentation Layer
- The presentation layer is the outermost layer of the iOS application and handles the user interface and presentation of output.
- This layer takes input from the users and translates data from the application layer into a suitable output.
- The components of the presentation layer depend on the technology stack and frameworks.
- The presentation layer works with the application layer through well-defined interfaces These interfaces separate the user interface from the business logic.
The core principle of these layers is the dependency rule, also known as the Dependency Inversion Principle (DIP). This rule says:
“All source code dependencies should point inwards.”
In a clean iOS architecture, the dependency inversion principle adds flexibility and scalability to the application. Since all the dependencies flow from high-level classes to low-level classes, it becomes easier to change the application’s components later on.
These were the 4 layers and dependency flow in a clean architecture in iOS. Now, let’s take a look at the principles of clean architecture to better understand the role of each layer.
5 Core Principles of Clean iOS Architecture
What distinguishes a clean architecture from a cluttered one? As per our software architecture team at Space-O Technologies, clean architecture has certain qualities that make it stand out. This section will shed light on all these qualities.
Independent of Frameworks
A clean architecture does not rely on external frameworks, libraries, or tools to function. Instead, the iOS application remains agnostic of implementation details and external dependencies. This way, the framework is implemented as a mere tool instead of a vital organ.
Independent of UI
In a clean iOS architecture, the user interface of the application changes seamlessly without making any changes to other layers. You could replace your iOS application’s UI with a web UI without making any changes to the core business logic, therefore promoting modularity.
Independent of Database
The business rules of a clean architecture are not bound to database implementation. This means separating business logic from the database, allowing programmers to shift the local database to any other technology at any time.
Independent of External Factors
The business rules of a clean architecture are isolated from external agencies. All the dependencies are managed and implemented independently. This way, a change in business logic does not necessitate changing external dependencies from third-party services.
Seamless Testability
The iOS developers can test the business logic, use cases, and external dependencies individually. No two layers are dependent on each other. Therefore, the testing team can test the app at different levels (unit test, integration test, and acceptance test.)
These were the fundamentals of creating an iOS app with a clean architecture. Now, let’s look at some of the patterns of clean architecture that can be used for developing iOS apps.
Top 3 Clean Architecture Patterns for iOS Development
Select the right clean architecture pattern to build a powerful iOS application. Here we have discussed three of the most popular clean architecture patterns for iOS- VIPER, MVVM, and Clean Swift (VIP).
VIPER
VIPER (View, Interactor, Presenter, Entity, and Router) is one of the most commonly used iOS development architectures. This architecture pattern helps developers write clean and concise codes that are divided into separate components based on their roles.
Each component of the program defines the data flow in the application. Let’s understand these components in detail.
- View: The View component represents the user interface of the application. This component receives user input and displays data to the user.
- Interactor: This component contains all the use cases for different scenarios and operations of the iOS application, from fetching information to performing operations.
- Presenter: The presenter is a mediator between the View and the Interactor. The presenter takes user input from the View, performs operations with the Interactor, and converts data into a presentable form for the user.
- Entity: Entity contains all the data structure and business logic of the iOS app. This information is usually stored in classes and structures.
- Router: The router is responsible for navigation and flow control. This component helps the user transition from one screen to the other.
MVVM
MVVM stands for Model-View-ViewModel and is another popular architecture pattern. The MVVM pattern entirely separates the user interface from the application logic. This pattern overcomes the shortages of other popular patterns like MVP and MVC.- Model: This component contains the business logic and data layers of the application. The Model abstracts data structures and validation rules of the program.
- View: View is the user interface component of the iOS program. View takes user input, informs ViewModel of the user’s action, and presents data to the users.
- ViewModel: ViewModel acts as an intermediary between View and Model and facilitates data binding. ViewModel interprets inputs from the View, updates the Model, and exposes relevant data streams to the View.
Want to know more about implementing the MVVM pattern in your iOS project? Check out this detailed guide on MVVM architecture for iOS development.
Clean Swift (VIP)
Clean Swift, a.k.a. VIP (View, Interactor, Presenter). Clean Swift offers ready-to-use Xcode templates to code your iOS application. These templates are neatly arranged and are easy to modify later on.- View Controller: The View Controller interacts with the user, captures their inputs, changes the UI based on changes in the data, and receives callbacks.
- Interactor: Interactor contains the business logic in your iOS app. This component can fetch and process data and even implement business rules.
- Presenter: The presenter acts as an intermediary between the View Controller and the Interactor. It takes input from the View Controller, processes it through the Interactor, and formats it to be given to the user.
- Model: Entities are data models within the iOS application. They define data structures, contain business objectives, and represent domain-specific concepts.
- Router: The Router decides which screen the user should go to next. This decision is based on the actions of the user and the output generated from app logic.
- Worker: This is an optional component that executes tasks not related to business logic per se. The Interactor may use this component for asynchronous or time-consuming tasks.
Now that you know about some of the top clean architecture patterns for iOS development, let’s take a look at some project examples. Doing so will help you understand how to execute your iOS code with a clean architecture.
Examples of Apps in Clean Architecture Patterns
Weather App in VIPER
- View: The View component in the weather forecast app will contain codes for displaying weather information. This component will also take the user’s inputs as commands.
Example: WeatherViewController.swift
import UIKit class WeatherVC: UIViewController, WeatherViewProtocol { let presenter: WeatherPresenterProtocol init (presenter: WeatherPresenterProtocol) { self .presenter = presenter self.init(nibName: "", bundle: nil) } required init? (coder: NSCoder ) { return nil } override func viewDidLoad () { super.viewDidLoad() setUI() } fileprivate func setUI () { // Implement UI setup here } } extension WeatherVC : UIViewController{ func handle(_ output: WeatherPresenterOutputs) { // Implement handling presenter outputs here } }
- Interactor: Here the interactor is responsible for fetching the weather data from the server and processing it for the application.
Example: WeatherInteractor.swift
final class WeatherInteractor: WeatherInteractorProtocol { weak var delegate: WeatherInteractorDelegate! }
- Presenter: The Presenter acts as an intermediary between the View Controller and Interactor. It will take commands from the user and process them using the Interactor.
Example: WeatherPresenter.swift
final class WeatherPresenter: WeatherPresenterProtocol { private let interactor: WeatherInteractorProtocol private let router: WeatherRouterProtocol weak var view: WeatherViewProtocol? init(_router: WeatherRouterProtocol, _ interactor: WeatherInteractorProtocol){ self.router = router self.interactor = interactor interactor.delegate = self } } extension WeatherPresenter: WeatherInteractorDelegate { func handle(_ output: WeatherInteractorOutputs) { } }
- Entities: This is the data model of the iOS application. In the context of a weather app, Entities will refer to the weather data that was previously fetched from the server.
Example: WeatherData.swift
//PRESENTER protocol WeatherPresenterProtocol: AnyObject { // Define presenter methods here } enum WeatherPresenterOutputs { case showError(String) case showData } //INTERACTOR protocol WeatherInteractorProtocol: AnyObject{ var delegate: WeatherInteractorDelegate? { get set } } protocol WeatherInteractorDelegate: AnyObject { func handle(_ output: WeatherInteractorOutputs) } enum WeatherInteractorOutputs { case sendError(String) case sendData } //ROUTER protocol WeatherRouterProtocol: AnyObject { var view: WeatherViewProtocol? { get set } func navigate(_ route: WeatherRoutes) } enum WeatherRoutes { } //VIEW protocol WeatherViewProtocol: AnyObject { func handle(_ output: WeatherPresenterOutputs) }
- Router: The Router will navigate the user from one screen of the weather app to the other. It will also decide on presenting and dismissing View Controllers.
Example: WeatherRouter.swift
import UIKit final class WeatherRouter: WeatherRouterProtocol { // MARK: - Properties weak var view: WeatherViewProtocol? // MARK: - Initialization init() { // Initialize any properties or dependencies here } // MARK: - Navigation func navigate(_ route: WeatherRoutes) { // Implement navigation logic here based on the route } }
To-do List App in MVVM
- Model: The Model layer in a to-do list app will contain a data structure that represents to-do items.
Example: TodoItem.swift
import Foundation struct TodoItem { var id: UUID var title: String var completed: Bool }
- View: This component in our to-do list app will display a list of to-do items to the user and will take their input. This input can be check-marking an item and adding/editing/removing an item.
Example: TodoListViewController.swift
import UIKit class TodoListViewController: UIViewController, TodoListViewModelDelegate { @IBOutlet weak var tableView: UITableView! var viewModel: TodoListViewModel! override func viewDidLoad() { super.viewDidLoad() // Initialize view model and set delegate viewModel = TodoListViewModel() viewModel.delegate = self // Fetch todo items viewModel.fetchTodoItems() } // Implement UITableViewDataSource and UITableViewDelegate methods to display to-do items func didFetchTodoItems() { // Implement logic to update UI with fetched todo items } }
- ViewModel: In a to-do app, the ViewModel component will fetch the list of to-do items from the Model component and display them in the View component.
Example: TodoListViewModel.swift
import Foundation protocol TodoListViewModelDelegate: AnyObject { func didFetchTodoItems() } class TodoListViewModel { weak var delegate: TodoListViewModelDelegate? var todoItems: [TodoItem] = [] func fetchTodoItems() { // Fetch to-do items from Model (e.g., database) delegate?.didFetchTodoItems() } // Other methods for updating, adding, or deleting to-do items }
Note-taking App in Clean Swift
- View Controller: This component will be responsible for displaying a list of notes to the user and capturing their inputs. These inputs can be adding/removing notes and formatting text.
Example: NotesViewController.swift
import UIKit protocol NotesDisplay: AnyObject { func displayNotes(viewModel: Notes.FetchNotes.ViewModel) } class NotesViewController: UITableViewController, NotesDisplay { var interactor: NotesBusinessLogic? var router: (NSObjectProtocol & NotesRoutingLogic & NotesDataPassing)? // MARK: Object lifecycle override init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: Bundle?) { super.init(nibName: nibNameOrNil, bundle: nibBundleOrNil) setup() } required init?(coder aDecoder: NSCoder) { super.init(coder: aDecoder) setup() } // MARK: Setup private func setup() { let viewController = self let interactor = NotesInteractor() let presenter = NotesPresenter() let router = NotesRouter() viewController.interactor = interactor viewController.router = router interactor.presenter = presenter presenter.viewController = viewController router.viewController = viewController router.dataStore = interactor } override func viewWillAppear(_ animated: Bool) { super.viewWillAppear(animated) let request = Notes.FetchNotes.Request() interactor?.fetchNotes(request: request) } func displayNotes(viewModel: Notes.FetchNotes.ViewModel) { } }
- Interactor: The Interactor will fetch and process notes from the central repository.
Example: NotesInteractor.swift
import UIKit protocol NotesBusinessLogic { func fetchNotes(request: Notes.FetchNotes.Request) } protocol NotesDataStore { var notes: [Notes]? { get } } class NotesInteractor: NotesBusinessLogic, NotesDataStore { var presenter: NotesPresentationLogic? var notes: [Notes]? func fetchNotes(request: Notes.FetchNotes.Request) { // Implement fetching notes logic here } }
- Presenter: The Presenter will take the inputs from the View Controller (edit, create, delete notes) and process them using the Interactor. This input will then be sent back to the View Controller as a result.
Example: NotesPresenter.swift
import UIKit protocol NotesPresentationLogic { func presentFetchedOrders(response: Notes.FetchNotes.Response) } class NotesPresenter: NotesPresentationLogic { weak var viewController: NotesDisplay? func presentFetchedOrders(response: Notes.FetchNotes.Response) { let displayedNotes: [Notes.FetchNotes.ViewModel.DisplayedNotes] = [] let viewModel = Notes.FetchNotes.ViewModel(displayedNotes: displayedNotes) viewController?.displayNotes(viewModel: viewModel) } }
- Model: The Model/Entity is the data model of the application. In a note-taking app, this model will be the note that the user adds to the application.
Example: Note.swift
import UIKit enum Notes { // MARK: Use cases enum FetchNotes { struct Request { // Request input for fetching notes } struct Response { var notes: [Note] } struct ViewModel { struct DisplayedNotes { var id: String var date: String var email: String var name: String var total: String } var displayedNotes: [DisplayedNotes] } } } struct Note { // Properties of a Note object // Add properties as needed }
- Router: The router will help the user navigate from one screen of the note-taking app to the other. The user can switch screens based on their commands and outputs.
Example: NotesRouter.swift
import UIKit @objc protocol NotesRoutingLogic { func notesCreate(segue: UIStoryboardSegue?) } protocol NotesDataPassing { var dataStore: NotesDataStore? { get } } class NotesRouter: NSObject, NotesRoutingLogic, NotesDataPassing { weak var viewController: NotesViewController? var dataStore: NotesDataStore? // MARK: Routing func notesCreate(segue: UIStoryboardSegue?) { } }
- Worker: The Worker component will fetch data from the repository to show to the user. This can include fetching pre-saved notes from the database.
Example: NotesWorker.swift
import UIKit class NotesWorker { func doSomeWork() { // This method can be used to perform tasks related to handling notes. // You can add functionality here to manipulate or process notes. // For example, saving notes to a database, fetching notes from a server, etc. } }
Clean Architectures in iOS: Take Your Pick
Clean architectures are becoming a go-to trend for iOS development. This architectural philosophy helps iOS developers create clean and concise codes for iOS applications. These codes are scalable, readable, testable, and easily modifiable.
All you need to do now is pick a clean architecture pattern that fits your application’s requirements. Still have questions? Check our FAQs to get more information.
Frequently Asked Questions
What is the use of clean architectures in iOS app development?
Implementing clean architecture principles makes your iOS code clean, readable, and editable. This way, you or any other developer can modify the app later on without having to browse through thousands of lines of code.
Is MVVM a clean architecture?
MVVM can be considered as a semi-clean architecture. While some components of the MVVM pattern align with clean architecture principles, this might not be the best choice for enterprise-level projects.
Which is the best architecture pattern for iOS?
VIPER is undoubtedly the best choice for clean iOS coding. Most iOS programmers prefer using VIPER components to program iOS applications.
Can I make an iOS app without following the clean architecture principles?
Yes, you can make an iOS app without following clean architecture principles. However, doing so is not recommended as architecture-less codes are challenging to debug and upgrade. You will have to spend time reading thousands of lines of code to identify errors and add functionalities. We therefore recommend you make your iOS app with clean architecture principles.
Get Our Expert’s Help for Clean Architecture Implementation
Do you still need help understanding clean architecture and how it can benefit your project? Are you looking for a way to implement clean architecture principles in your iOS development project? Contact us today.
Space-O Technologies has a team of industry-best software architects with proven experience in implementing clean architecture principles. We have the right expertise to improve your project’s efficiency and quality through clean architecture implementation.