The Model-View-ViewModel (MVVM) pattern is essential for structuring iOS apps in Swift, making code clean, easy to manage, and scalable. It separates your app into three parts: the View for the user interface, the Model for the data, and the ViewModel for the logic that connects them.
This setup boosts maintainability and testing. If you’re starting with iOS MVVM architecture or need expert help, consider partnering with our iOS app development company. We excel in applying MVVM to enhance app development. Let’s explore how to effectively implement MVVM in your Swift projects.
Contents
What is MVVM Architecture?
Model-View-ViewModel (MVVM) architecture is a design pattern that separates business logic from UI components. This separation results in a more organized code structure which ultimately leads to improved maintainability and scalability. MVVM is also known as model-view-binder and was created by Microsoft architects Ken Cooper and John Gossman.
Like many other design patterns, MVVM organizes code and breaks programs into modules to make the development, updating, and reuse of code simpler and faster. The MVVM pattern is used in Windows Presentation Foundation (WPF), which runs on Microsoft. NET.
The separation code in MVVM is divided into View, ViewModel , and Model:
- View: This is the part of the app you see and interact with, like the screens and forms.
- ViewModel: It acts as a middleman that takes care of what happens when you interact with the View. It prepares the data so that you can see it and decides what to do when you click buttons.
- Model: This is where the app’s main work happens. It deals with the data—storing, retrieving, and everything in between. It’s all about the logic that makes the app function as intended.
So, by dividing an application into these three components, MVVM makes your code more organized and adaptable, simplifying the development of complex applications.
What does it mean when we say clean code?
Here is an example.
//MODEL
import Foundation
struct User: Codable, Identifiable {
var id: String {
return nama! // Assuming nama is non-optional and acts as the ID
}
let nama: String? // Name of the user
let lokasi: String? // Location of the user
let deskripsi: String? // Description of the user
let thumbnail: String? // URL string for the user's thumbnail image
let gambar: String? // URL string for the user's main image
}
//VIEW
import SwiftUI
struct Profile: View {
@ObservedObject var viewModel = ProfileViewModel()
var body: some View {
NavigationView {
ZStack {
List {
ForEach(viewModel.user) { user in
VStack(alignment: .leading) {
Text(user.nama ?? "Unknown Name")
.font(.headline)
Text(user.lokasi ?? "Unknown Location" )
.font(.subheadline)
.foregroundColor(.gray)
Text(user.deskripsi ?? "No Description")
}
}
}
.listStyle(.plain)
}
.navigationBarTitle(Text("List Place"), displayMode: .inline)
}
.navigationBarHidden(true)
.onAppear(perform: {
viewModel.fetchUsers()
})
}
}
//VIEWMODEL
import Foundation
class ProfileViewModel: ObservableObject {
@Published var user = [User]()
private let callapi = APIServices()
func fetchUsers() {
callapi.fetchPlaces { response in
if (response.error == nil) {
self.user = response.value ?? []
} else {
print("error \(response.error!)")
}
}
}
}
Looking for iOS App Development?
Let’s talk. We have iOS app developers who are experts in using MVVM architecture to develop your solution with clean code.
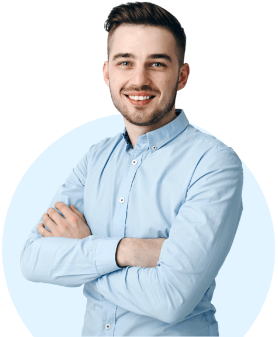
Now, let’s understand the benefits of using MVVM in iOS development.
Why Use MVVM in iOS Development?
Here are the advantages of MVVM architecture in Swift programming language.
Enhanced Code Organization
MVVM separates UI (View) from business logic (Model), leading to cleaner, more manageable code. This means the parts of your app that handle what it looks like are kept separate from the parts that handle what it does.
For example: In a note-taking app, the ViewModel can provide formatted note previews for the View to display, separating the display logic from the Model that handles note storage and retrieval. This division simplifies the overall codebase by organizing it into distinct layers.
Easier Testing
Because the UI and business logic are separate, testing the app’s functionality (via the ViewModel) doesn’t require UI elements, making tests simpler and more reliable.
For Example: For a weather app, you can test the ViewModel to ensure it correctly formats temperature data fetched from the Model without needing to interact with the View. This makes tests faster and less prone to breakage from UI changes.
Dynamic UIs with Data Binding
The pattern supports data binding and reactive programming, allowing automatic UI updates when data changes and vice versa, leading to lively, responsive user interfaces.
Example: A stock market app automatically updates stock prices on the View as they change. The ViewModel binds these price updates from the Model to the View, so the user sees real-time data without manual refreshes.
Independent Workflows
Designers and developers can work more independently thanks to the clear division of UI design and logic development, improving efficiency and speeding up the development process.
For example: A design team can work on the app’s UI (View) independently from the developers who focus on the data logic (Model) and presentation logic (ViewModel). This parallel workflow speeds up development time.
Maintenance Ease
MVVM’s modular nature makes it easier to update or add features without risking other parts of the app, minimizing the chance of new bugs.
Example: Adding a new feature, like a search function, primarily involves updates to the ViewModel and View for the UI elements, without altering the Model. This localized change minimizes risk and makes the feature easier to implement.
Quick UI Adaptability
The architecture facilitates easy UI changes or overhauls without needing to alter the underlying business logic, keeping the app modern with less effort.
Example: If the UI of a fitness tracking app needs a redesign for a more modern look, changes can be made to the View without affecting the Model that tracks the fitness data or the ViewModel that processes it, ensuring a smooth transition.
Swift and SwiftUI Synergy
MVVM aligns well with Swift and SwiftUI, particularly with their data binding and declarative approaches, enhancing app development in modern iOS environments.
Example: SwiftUI’s declarative syntax works well with MVVM, as data can be bound from the ViewModel to the View with minimal boilerplate code, making development faster and more intuitive with Swift’s modern features.
Since we have studied the benefits, let’s check how MVVM is different from MVC and MVP.
Want to Transform Your Application Architecture?
Contact us for a personalized consultation on implementing MVVM architecture in your projects.
How is MVVM Different From Other Design Patterns Like MVC and MVP?
Here is a table showcasing a detailed comparison of how MVVM stands out
Aspects | MVVM | MVC | MVP |
---|---|---|---|
Decouples UI and Logic | |||
Supports Two-way Data Binding | – | – | |
High Testability | – | ||
Minimal UI Layer Dependency | – | – | |
Simplifies Codebase | – | – | |
Facilitates Dynamic UI Updates | – | – | |
Improves Collaboration Between Developers and Designers | – | ||
Adaptable to UI Changes Without Affecting Business Logic | – | – | |
Clear Separation of Concerns | |||
Well-defined Framework Support |
Let’s understand some of the considerations taken care to develop a solution using MVVM architecture.
Key Considerations When Implementing the MVVM Architecture in iOS
Data Binding: Essential for MVVM, but iOS doesn’t offer it out of the box. Tools like Combine can help bridge this gap.
ViewModels: This should be lean and independent from UI elements, ensuring they’re test-friendly and versatile.
Handling State: It’s crucial to manage app states (loading, error, etc.) efficiently in ViewModels for a smooth user experience.
Use Dependency Injection: This keeps ViewModels flexible by not hard-wiring them to specific models, aiding in testing and reusability.
Respect Layers: Keep a clean separation between the Model, View, and ViewModel layers to maintain order and clarity.
Focus on Testability: MVVM shines here; structure your code to easily test ViewModels independently.
Watch Performance: Overdoing data binding or observers can slow things down. Keep it efficient.
Mind the Learning Curve: MVVM can be complex for newcomers. Provide resources and time for your team to adapt.
SwiftUI and MVVM: They go well together. Understand how to use SwiftUI’s features to complement MVVM’s strengths.
Want to Develop a Custom iOS Application?
Contact us. Our iOS developers develop high-quality mobile apps tailored to your business needs and requirements.
How to Test Your Implementation Of the MVVM Architecture Pattern in iOS
ViewModel Testing
- Mock the Model: Use mock objects for the data model to simulate real data and scenarios.
- Test Data Binding: Ensure that changes in the ViewModel are correctly propagated to the View through data binding.
- Test Commands: Verify that actions (commands) initiated from the View are executed as expected.
- Test State Management: Check how the ViewModel handles different states, like loading, error, and success.
Objective: Test the business logic and presentation logic within the ViewModel without UI dependencies.
Approach:
Model Testing
- Use unit tests to validate the logic and operations within your models, such as data validation, transformations, and business rules.
- Mock external dependencies, such as network requests or database access, to ensure tests are not dependent on external factors.
Objective: Test the data handling and business logic encapsulated in the Model.
Approach:
View Testing
- Use UI tests to verify the user interface behaves as expected when interacting with the ViewModel.
- Test dynamic UI changes triggered by ViewModel updates, ensuring the UI correctly reflects ViewModel states.
Objective: While the View is usually tested via UI tests, MVVM aims to minimize logic in the View, so the focus here is on layout and user interactions.
Approach:
Integration Testing
- Simulate real-world use cases to ensure the ViewModel interacts with the Model correctly and updates the View accordingly.
- Test the complete flow from user input through the ViewModel to the Model and back to the View.
Objective: Verify the interaction between components, especially between ViewModel and Model.
Approach:
Tools and Frameworks Used to Test MVVM Architecture Pattern in iOS
brief overview of each and how they apply to testing different aspects of MVVM:
- XCTest: Apple’s testing framework that supports both unit and UI tests.
- Mocking Libraries: Use libraries like Cuckoo or SwiftyMocky to generate mock objects for testing.
- Combine: If using Combine for data binding, test publishers and subscribers to ensure data flows as expected.
3 Best Practices to Test MVVM Architecture
- Continuous Integration (CI): Integrate testing into your CI pipeline to catch issues early.
- Test Coverage: Aim for high test coverage but focus on testing the logic and scenarios that are most critical to your app’s functionality.
- Documentation: Document test cases and scenarios to maintain clarity and ensure important edge cases are covered.
By following this approach, you can ensure your MVVM implementation in iOS is robust, maintainable, and functions as intended, facilitating easier updates and scalability.
Common Mistakes When Implementing MVVM Architecture Patterns and How to Avoid Them
Implementing the MVVM architecture pattern can streamline and improve your development process, but like any architectural pattern, there are common pitfalls to be aware of. Understanding these pitfalls and knowing how to avoid them can help you leverage MVVM effectively. Here are some common mistakes and tips for avoiding them:
Overcomplicating the ViewModel
Mistake: Packing too much logic or too many responsibilities into the ViewModel.
Solution: Keep the ViewModel focused on UI logic and state management. Use services for data fetching and business logic to keep the ViewModel clean and maintainable.
Direct References to View Elements in ViewModel
Mistake: Creating direct references to UI elements within the ViewModel, which breaks the separation of concerns.
Solution: Ensure the ViewModel only exposes data and commands. Use data binding to connect the View and ViewModel without direct references.
Ignoring the Model
Mistake: Neglecting the Model’s design, leading to a bloated ViewModel as it takes on responsibilities that should belong to the Model.
Solution: Design a robust Model that handles data manipulation, validation, and business logic, ensuring the ViewModel remains streamlined.
Not Utilizing Data Binding
Mistake: Failing to implement data binding effectively, resulting in manual management of UI updates.
Solution: Use data binding to automatically update the UI when the data state changes in the ViewModel. Explore frameworks that support data binding if your development environment doesn’t provide robust options.
Testing Neglect
Mistake: Not prioritizing testing, especially for the ViewModel, or testing it inadequately.
Solution: Take advantage of the testability of the MVVM pattern by writing thorough unit tests for the ViewModel, focusing on its interaction with the Model and its data handling.
Misunderstanding MVVM’s Role
Mistake: Misapplying MVVM or using it where it may not be necessary, leading to unnecessary complexity.
Solution: Understand the strengths and weaknesses of MVVM. Apply it in projects where its advantages in separation of concerns, testability, and maintainability are beneficial.
Lack of Layered Architecture Discipline
Mistake: Allowing tight coupling between layers, can lead to a monolithic design that’s hard to maintain and test.
Solution: Strictly adhere to the MVVM structure, ensuring clear boundaries between the Model, View, and ViewModel. Use dependency injection to further decouple components.
Ignoring Performance Implications
Mistake: Overusing observables or not managing subscriptions efficiently, leading to memory leaks and performance issues.
Solution: Be mindful of the lifecycle of observables and subscriptions. Dispose of them properly to avoid memory leaks. Optimize data binding to prevent unnecessary updates.
By recognizing these common mistakes and applying the suggested solutions, iOS developers can more effectively implement the MVVM pattern, leading to cleaner, more maintainable, and scalable applications.
Ready to Use MVVM for Your Next Project?
MVVM architecture simplifies testing and makes your app adaptable to UI changes with minimal impact on the underlying business logic. MVVM’s compatibility with Swift and SwiftUI streamlines to build responsive applications. By maintaining a disciplined structure and focusing on the core advantages of MVVM—testability, modularity, and a clear separation of concerns—you set the stage for building scalable iOS applications. Ready to start on your next project with MVVM? With these insights, you can make the most of this powerful architectural pattern.